Module docusign.dsclick
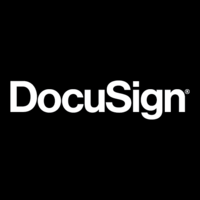
ballerinax/docusign.dsclick Ballerina library
Overview
DocuSign is a digital transaction management platform that enables users to securely sign, send, and manage documents electronically.
The Ballerina DocuSign Click connector integrates with the DocuSign platform, provides APIs to capture user consent with one click for simple agreements such as terms & conditions and privacy policies within Ballerina applications. It supports DocuSign Click API V2.
Setup guide
To utilize the DocuSign Click connector, you must have access to the DocuSign REST API through a DocuSign account.
Step 1: Create a DocuSign account
In order to use the DocuSign Click connector, you need to first create the DocuSign credentials for the connector to interact with DocuSign.
-
You can create an account for free at the Developer Center.
Step 2: Create integration key and secret key
-
Create an integration key: Visit the Apps and Keys page on DocuSign. Click on
Add App and Integration Key,
provide a name for the app, and clickCreate App
. This will generate anIntegration Key
. -
Generate a secret key: Under the
Authentication
section, click onAdd Secret Key
. This will generate a secret Key. Make sure to copy and save both theIntegration Key
andSecret Key
.
Step 3: Generate refresh token
-
Add a redirect URI: Click on
Add URI
and enter your redirect URI (e.g., http://www.example.com/callback). -
Generate the encoded key: The
Encoded Key
is a base64 encoded string of yourIntegration key
andSecret Key
in the format{IntegrationKey:SecretKey}
. You can generate this in your web browser's console using thebtoa()
function:btoa('IntegrationKey:SecretKey')
. You can either generate the encoded key from an online base64 encoder. -
Get the authorization code: Visit the following URL in your web browser, replacing
{iKey}
with your Integration Key and{redirectUri}
with your redirect URI.https://account-d.docusign.com/oauth/auth?response_type=code&scope=signature%20organization_read%20click.manage&client_id={iKey}&redirect_uri={redirectUri}
This will redirect you to your redirect URI with a
code
query parameter. This is yourauthorization code
. -
Get the refresh token: Use the following
curl
command to get the refresh token, replacing{encodedKey}
with your Encoded Key and{codeFromUrl}
with yourauthorization code
.curl --location 'https://account-d.docusign.com/oauth/token' \ --header 'Authorization: Basic {encodedKey}' \ --header 'Content-Type: application/x-www-form-urlencoded' \ --data-urlencode 'code={codeFromUrl}' \ --data-urlencode 'grant_type=authorization_code'
The response will contain your refresh token. Use
https://account-d.docusign.com/oauth/token
as the refresh URL.
Remember to replace {IntegrationKey:SecretKey}
, {iKey}
, {redirectUri}
, {encodedKey}
, and {codeFromUrl}
with your actual values.
Above is about using the DocuSign Click APIs in the developer mode. If your app is ready to go live, you need to follow the guidelines given here to make it work.
Quickstart
To use the DocuSign Click connector in your Ballerina project, modify the .bal
file as follows.
Step 1: Import the module
Import the ballerinax/docusign.dsclick
module into your Ballerina project.
import ballerinax/docusign.dsclick;
Step 2: Instantiate a new connector
Create a dsclick:ConnectionConfig
with the obtained OAuth2.0 tokens and initialize the connector with it.
configurable string clientId = ?; configurable string clientSecret = ?; configurable string refreshToken = ?; configurable string refreshUrl = ?; dsclick:Client docuSignClient = check new({ auth: { clientId, clientSecret, refreshToken, refreshUrl } });
Step 3: Invoke the connector operation
You can now utilize the operations available within the connector.
public function main() returns error? { // Prepare the clickwrap request payload dsclick:ClickwrapRequest returnPolicyPayload = { clickwrapName: "ReturnPolicy", documents: [ { documentName: "Test Doc", documentBase64: "base64-encoded-pdf-file", fileExtension: "pdf" } ], displaySettings: { displayName: "Return Policy", consentButtonText: "I Agree", downloadable: true, format: "modal", requireAccept: true, documentDisplay: "document", sendToEmail: true } }; // Create a new clickwrap dsclick:ClickwrapVersionSummaryResponse newClickWrap = check docuSignClient->/v1/accounts/[accountId]/clickwraps.post(returnPolicyPayload); }
Hint: To apply a value to the
documentBase64
field, you can either use an online tool designed to convert a PDF file into a base64-encoded string, or you can refer to the provided example code
Step 4: Run the Ballerina application
Use the following command to compile and run the Ballerina program.
bal run
Examples
The DocuSign Click connector provides practical examples illustrating usage in various scenarios. Explore these examples.
-
Managing return policy agreement clickwrap with DocuSign This example shows how to use DocuSign Click API to to implement a clickwrap agreement for a return policy to ensure customers acknowledge and agree to the terms before making a purchase.
-
Managing terms and conditions clickwrap with DocuSign This example shows how to use DocuSign Click API to to implement a clickwrap agreement for a terms and condition application and users can agree them with just one click.
Import
import ballerinax/docusign.dsclick;
Other versions
2.0.0